Mocking HttpClient in .Net Core
- Sudherson Vetrichelvan
- Aug 18, 2020
- 1 min read
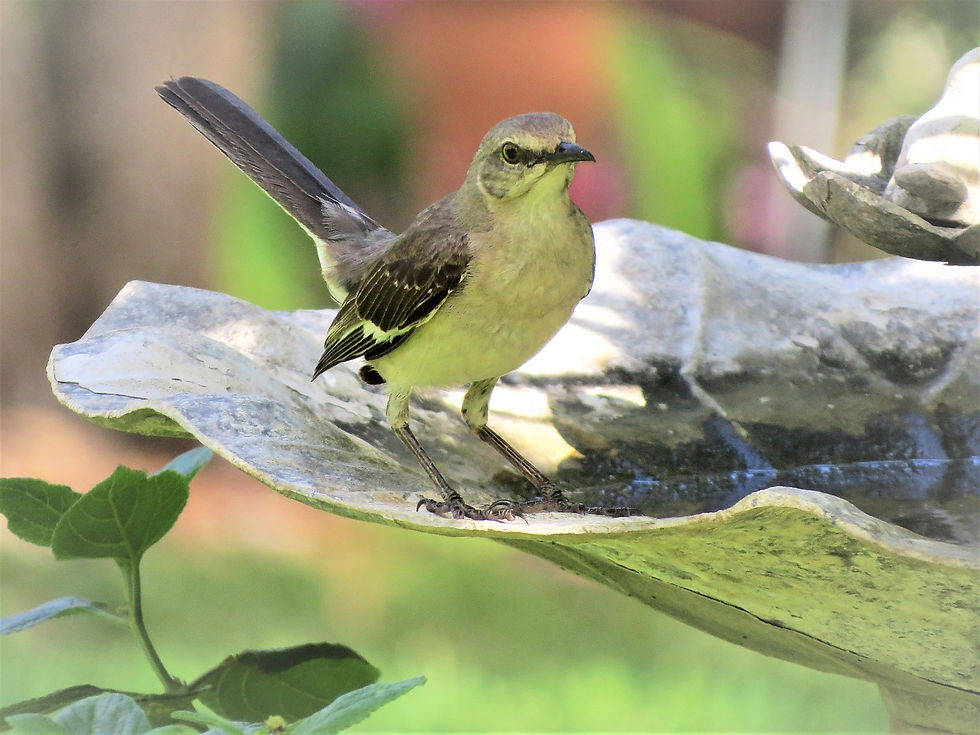
There's inbuilt support to apply conditions on HttpMethod and RequestUri properties of HttpRequestMessage. This way we can mock HttpGet, HttpPost and other verbs for various paths using the EndsWith method as described below.
using System.Net; using System;
using System.Threading;
using System.Net.Http;
using System.Threading.Tasks;
using System.Net.Http.Formatting;
using NUnit.Framework;
using Moq; using Moq.Protected;
public class HttpServiceTests { private readonly IHttpService _service; private readonly Mock<HttpMessageHandler> _httpMessageHandler = new Mock<HttpMessageHandler>(); private readonly Mock<HttpClient> _httpClient = new Mock<HttpClient>();
public HttpServiceTests() { var _httpClient = new HttpClient(_httpMessageHandler.Object); _service = new HttpService(_configuration, _httpClient); }
[SetUp] public void Setup() { _httpMessageHandler.Protected() .Setup<Task<HttpResponseMessage>>("SendAsync", true,
// Specify conditions for httpMethod and path ItExpr.Is<HttpRequestMessage>(req => req.Method == HttpMethod.Get && req.RequestUri.AbsolutePath.EndsWith($"{path}"))), ItExpr.IsAny<CancellationToken>()) .ReturnsAsync(new HttpResponseMessage { StatusCode = HttpStatusCode.OK, Content = new StringContent("_0Kvpzc") }); _httpMessageHandler.Protected() .Setup<Task<HttpResponseMessage>>("SendAsync",
// Specify conditions for httpMethod and path ItExpr.Is<HttpRequestMessage>(req => req.Method == HttpMethod.Post && req.RequestUri.AbsolutePath.EndsWith($"{path}")), ItExpr.IsAny<CancellationToken>()) .ReturnsAsync(new HttpResponseMessage { StatusCode = HttpStatusCode.OK, Content = new ObjectContent<T>(new T { Prop1 = "value1", Prop2 = "value2", }, new JsonMediaTypeFormatter()) }); }
/// <summary> /// Http Get successful /// </summary> [Test] public async Task HttpGet_Success() { var response = await _service.Get("path1"); }
/// <summary> /// Http Post returns 401 /// </summary> [Test] public async Task HttpPost_Returns_401() { _httpMessageHandler.Protected() .Setup<Task<HttpResponseMessage>>("SendAsync",
// Override conditions for httpMethod and path specific to test ItExpr.Is<HttpRequestMessage>(req => req.Method == HttpMethod.Post && req.RequestUri.AbsolutePath.EndsWith($"{path3}")), ItExpr.IsAny<CancellationToken>()) .ReturnsAsync(new HttpResponseMessage { StatusCode = HttpStatusCode.Unauthorized, Content = new StringContent("Unauthorized") }); var response = await _service.Get("path2"); } }